1
This post will serve as a guide for anyone interested in learning web development. With it, you will be able to have a reference of which path to follow to learn frontEnd and backEnd. Below is a complete roadmap to guide you in your learning.
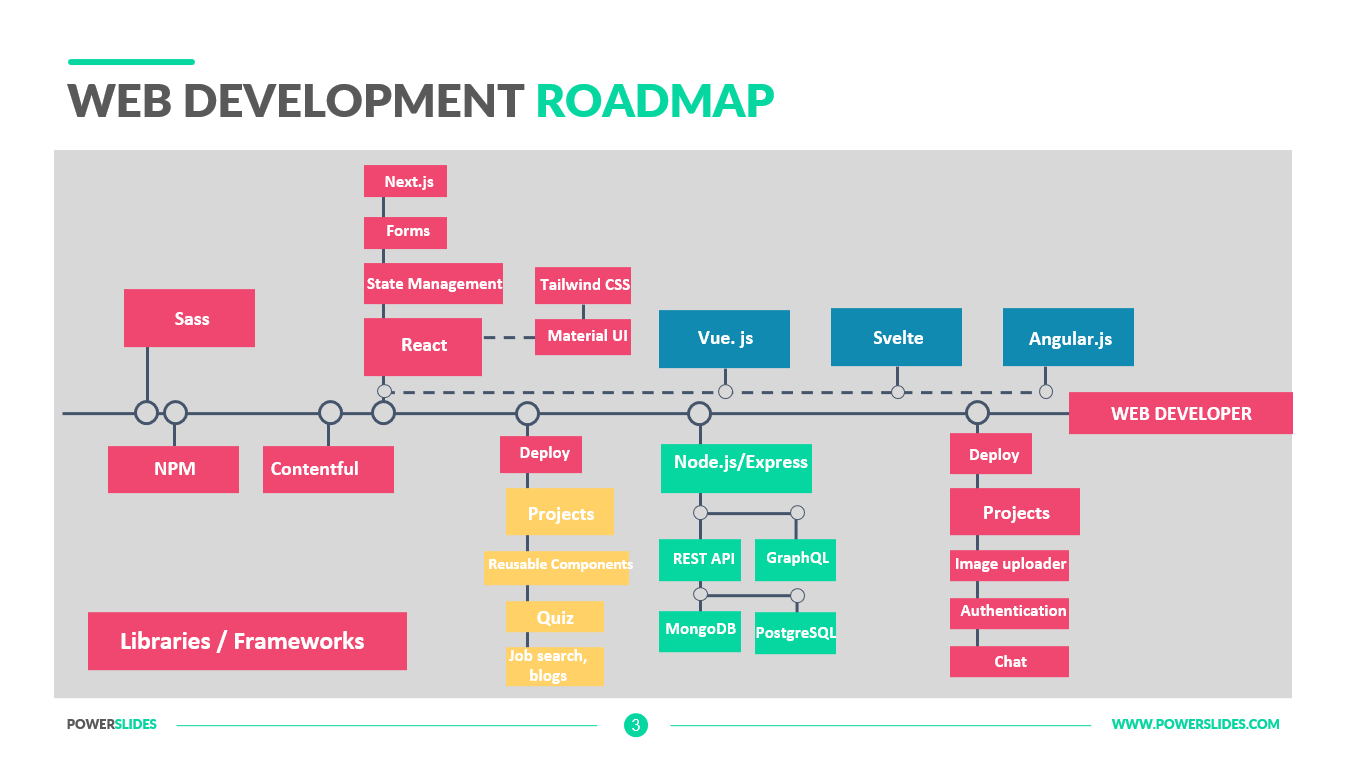
## 1. Web Fundamentals
### HTML
- [x] Basic HTML structure and syntax
- [x] Semantic HTML elements
- [x] Forms and input elements
- [x] HTML5 features (e.g., audio, video, canvas)
### CSS
- [x] CSS selectors and specificity
- [x] Box model and layout techniques
- [x] Flexbox and Grid for modern layouts
- [x] Responsive design and media queries
- [x] CSS preprocessors (e.g., Sass, Less)
### JavaScript (JS)
- [x] Variables, data types, and operators
- [x] Control flow and loops
- [x] Functions and scope
- [x] DOM manipulation
- [x] Events and event handling
## 2. Advanced Frontend Development
### Intermediate JavaScript
- [ ] ES6+ features (e.g., arrow functions, destructuring)
- [ ] Arrays and array methods (e.g., map, filter, reduce)
- [ ] Asynchronous JavaScript and Promises
- [ ] Modules and module bundlers (e.g., Webpack, Parcel)
### Frontend Framework - React
- [ ] Basics of React (components, props, state)
- [ ] JSX syntax and expressions
- [ ] Handling forms and user input
- [ ] React Router for client-side routing
- [ ] State management with Redux or Context API
### CSS Frameworks and Libraries
- [ ] Bootstrap for rapid UI development
- [ ] Material-UI or Ant Design for React component libraries
- [ ] Styled-components for styling React components with CSS-in-JS
## 3. Backend Development
### Node.js and Express
- [ ] Basics of Node.js and its ecosystem
- [ ] Setting up a server with Express.js
- [ ] RESTful API design and implementation
- [ ] Middleware and error handling
- [ ] Authentication and authorization with Passport.js
### Databases
- [ ] Basics of SQL and relational databases
- [ ] NoSQL databases (e.g., MongoDB) and document-oriented data modeling
- [ ] ORM/ODM libraries (e.g., Sequelize, Mongoose)
### Deployment and DevOps
- [ ] Version control with Git and GitHub
- [ ] Deploying web applications to platforms like Heroku, Netlify, or Vercel
- [ ] Continuous integration and deployment (CI/CD) pipelines
- [ ] Monitoring and logging tools (e.g., Sentry, New Relic)
## 4. Full Stack Development
### Integrating Frontend with Backend
- [ ] Consuming RESTful APIs in React
- [ ] Handling authentication and authorization in a full-stack application
- [ ] Optimizing performance and security
### Advanced React and State Management
- [ ] React Hooks for functional components
- [ ] Context API for global state management
- [ ] Advanced patterns (e.g., higher-order components, render props)
- [ ] Testing React applications (unit testing, integration testing)
### GraphQL and Apollo Client
- [ ] Basics of GraphQL and its advantages over REST
- [ ] Setting up a GraphQL server with Apollo Server
- [ ] Querying data with Apollo Client in React applications
- [ ] Real-time data with subscriptions
## 5. Continuous Learning and Specialization
### Advanced Topics
- [ ] Server-side rendering (SSR) with Next.js or Gatsby
- [ ] Websockets for real-time communication
- [ ] Performance optimization techniques (code-splitting, lazy loading)
- [ ] Progressive Web Apps (PWAs) for offline access and improved performance
### Specialization Tracks
- [ ] Frontend specialization (e.g., UX/UI design, accessibility)
- [ ] Backend specialization (e.g., microservices architecture, serverless computing)
- [ ] DevOps and infrastructure (e.g., Docker, Kubernetes, AWS)
### Professional Development
- [ ] Building a portfolio of projects
- [ ] Contributing to open-source projects
- [ ] Participating in hackathons and coding competitions
- [ ] Networking and attending tech events and conferences
If you'd like to learn about this guide for free, come and talk to me [here](https://chat-to.dev/chat?q=javascript-room), and don't forget to leave your comment here, as this is very important to me. Thank you
This post will serve as a guide for anyone interested in learning web development. With it, you will be able to have a reference of which path to follow to learn frontEnd and backEnd. Below is a complete roadmap to guide you in your learning.
You must log in or register to comment.